Here we are discussing simple concept for create custom Block and render on template. Just simple Steps, Please follow this.
1. We have to create folder under "/modules/custom/herobanner".
2. Inside this 'myblock' folder have to create 'info.yml' file like..
Example:
herobanner.info.ymlname: Hero Banner
type: module
description: Homepage hero banner.
core: '8.x'
package: Custom
dependencies:
- block 3. Then we have to create one more folder like "/modules/herobanner/src/Plugin/Block" and create file in this folder with name of "HerobannerBlock.php".
There we will mention "Annotation meta data", that will allow us to identify the Block.
Here "HerobannerBlock" class will contain
4 methods:build(), blockAccess(), blockForm(), blockSubmit()4. Now we will start code in "HerobannerBlock.php" file.
<?phpnamespace Drupal\herobanner\Plugin\Block;
use Drupal\Core\Access\AccessResult;
use Drupal\Core\Block\BlockBase;
use Drupal\Core\Form\FormStateInterface;
use Drupal\Core\Session\AccountInterface;
use Drupal\Core\Link;
/**
* Provides a 'Banner' Block
*
* @Block(
* id = "banner_block",
* admin_label = @Translation("Banner block"),
* )
*/class HerobannerBlock extends BlockBase {
/**
* {@inheritdoc}
*/ public function build() {
return [
'#markup' => $this->t('This is a custom block example.'),
];
}
/**
* {@inheritdoc}
*/ protected function blockAccess(AccountInterface $account) {
return AccessResult::allowedIfHasPermission($account, 'access content');
}
/**
* {@inheritdoc}
*/ public function blockForm($form, FormStateInterface $form_state) {
$config = $this->getConfiguration();
return $form;
}
/**
* {@inheritdoc}
*/ public function blockSubmit($form, FormStateInterface $form_state) {
$this->configuration['herobanner_settings'] = $form_state->getValue('herobanner_settings');
}
}
Example:
namespace Drupal\herobanner\Plugin\Block;
use Drupal\Core\Access\AccessResult;
use Drupal\Core\Block\BlockBase;
use Drupal\Core\Form\FormStateInterface;
use Drupal\Core\Session\AccountInterface;
use Drupal\Core\Link;
/**
* Provides a 'Banner' Block
*
* @Block(
* id = "banner_block",
* admin_label = @Translation("Banner block"),
* )
*/
class HerobannerBlock extends BlockBase {
/**
* {@inheritdoc}
*/
public function build() {
/**
* Read homepage_banner_products homepage_hero_banner
*/
$query = \Drupal::entityQuery('taxonomy_term');
$query->condition('vid', "homepage_banner_products");
$query->sort('weight','ASC');
$tids = $query->execute();
$homepage_banner_products_terms = \Drupal\taxonomy\Entity\Term::loadMultiple($tids);
$i=0;
$product_slider = '';
foreach ($homepage_banner_products_terms as $term) {
$product_image_path = $term->field_image->entity->getFileUri();
$img_url = file_create_url($product_image_path);
$product_image = $term->get('field_image')->getValue();
$prdct_alt = $product_image[0]['alt'];
$new_window = $term->get('field_open_in_new_window')->getValue();
$target = (isset($new_window[0]) && $new_window[0]['value'] == 1)?'target="_blank"':'';
$enddate = $term->get('field_end_date')->getValue();
$curdate = date('Y-m-d');
if($enddate >= $curdate){
$active_class = ($i == 0)?'active':'';
$product_slider.='<div class="item '.$active_class.'">
<div class="item-inner">
<h2>'.$term->name->value.'</h2>
<div class="productthumb"><img src="'.$img_url.'" alt="'.$prdct_alt.'"></div>
'.$term->description->value.'
<a href="'.$term->field_title->value.'" '.$target.' class="linkBtn mdhbanner">'.$term->field_support_option_title->value.'<span class="icon-icon_link"></span></a>
</div>
</div>';
$i++;
}
}
$html = '<div class="arc-container layer-3 layer" data-depth=".5" data-type="parallax">
<div class="arc-circle">
<div class="featuredproduct">
<div class="pop-scroll-outer">
<div class="pop-scroll">
<div class="slidercontrol">
<a type="button" class="slick-prev slickbutton carousel-control" href="javascript:void(0)">
<span class="icon-icon_link icon-flip" href="#myCarousel" data-slide="prev"></span>
</a>
<strong>Featured Products</strong> <span class="num"></span>
<a type="button" class="slick-next slickbutton carousel-control" href="javascript:void(0)">
<span class="icon-icon_link" href="#myCarousel" data-slide="next"></span>
</a>
</div>
<div id="myCarousel" class="carousel slide" data-ride="carousel" data-interval="false">
<div class="carousel-inner">
'.$product_slider.'
</div>
</div>
</div>
</div>
<!--Featured Product-->
</div>
</div>
</div>
</div>';
return array(
'#markup' => $this->t($html),
);
}
}?>Now we have to install and Enable our module and then clear the cache.
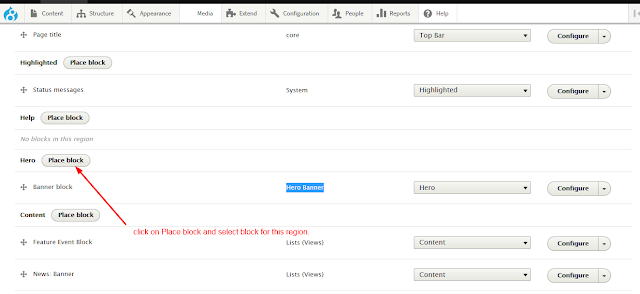 |
Place Block |
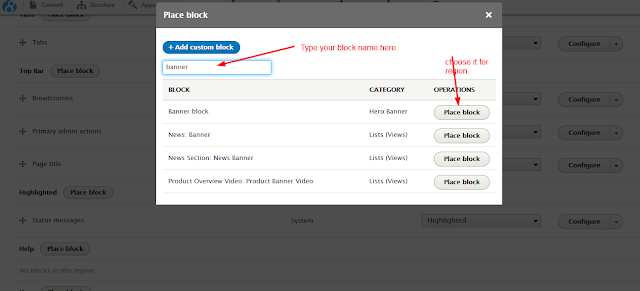 |
Choose Block |
And Pass the '
id' of your Block in this function..
Syntax: {{ drupal_block('myblock_id') }}
Example: {{ drupal_block('banner_block') }}After this clear the cache and get the result.
Note:
If have any suggestions or issue regarding 'How to Create Custom Block and Render on template in drupal 8?' then you can ask by
comments.